Frontend vs Backend
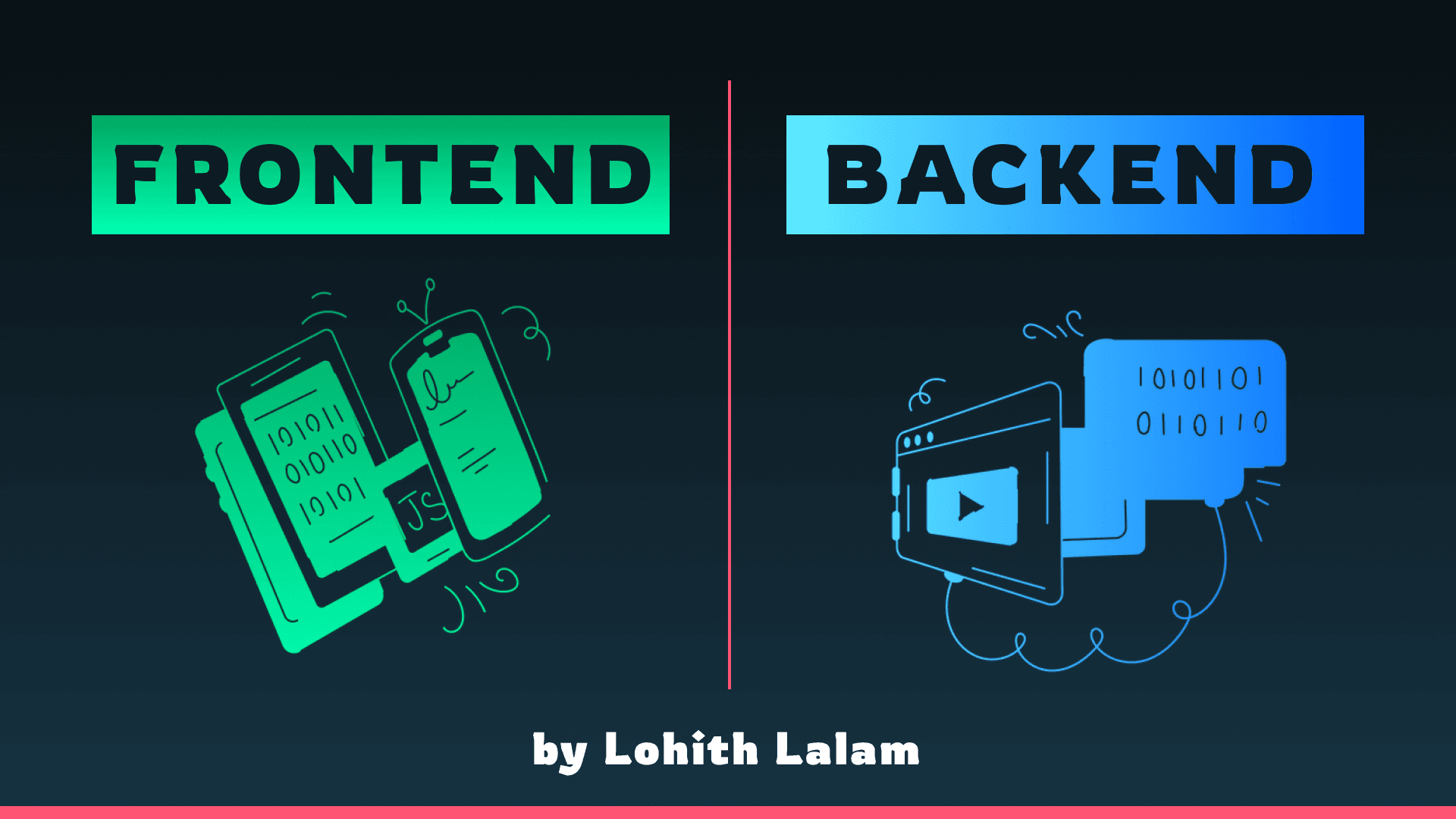
Web development is a multifaceted field, involving various technologies and processes to create functional, aesthetically pleasing, and efficient websites and web applications. Central to this process are two critical components: the frontend and the backend. Each plays a distinct yet interconnected role in the development cycle. This article delves into the significance of both frontend and backend development, highlighting their differences, interdependencies, unique contributions to the overall user experience, and the resources required for each.
Frontend Development: The Face of the Web
1. User Interface and User Experience
Frontend development, often referred to as client-side development, is primarily concerned with everything that users interact with directly. This includes the layout, design, and interactivity of a website. The goal is to ensure that users have a seamless, intuitive, and engaging experience.
- Languages and Tools: HTML, CSS, and JavaScript are the core technologies used in frontend development. Frameworks and libraries like React, Angular, and Vue.js further enhance the capabilities of these languages, allowing developers to create dynamic and responsive interfaces.
- Design and Usability: A significant part of frontend development involves working closely with designers to implement visually appealing and user-friendly interfaces. This encompasses everything from the color scheme and typography to navigation and accessibility features.
<!-- Sample HTML code block -->
<!DOCTYPE html>
<html>
<head>
<title>My Website</title>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a sample paragraph.</p>
<button onclick="displayAlert()">Click Me</button>
<script>
function displayAlert() {
alert('Button clicked!');
}
</script>
</body>
</html>
2. Performance and Responsiveness
Frontend developers also focus on optimizing the performance of a website. This includes ensuring fast load times, smooth scrolling, and responsiveness across different devices and screen sizes.
- Responsive Design: Techniques such as responsive design ensure that websites adapt seamlessly to various devices, providing an optimal viewing experience on desktops, tablets, and smartphones.
/* Sample CSS code block */
body {
font-family: Arial, sans-serif;
}
h1 {
color: #333;
}
@media (max-width: 600px) {
h1 {
font-size: 24px;
}
}
- Performance Optimization: Frontend developers use various strategies to enhance performance, including minimizing code, optimizing images, and leveraging browser caching.
3. Resources Required for Frontend Development
- Code Editors: Tools like Visual Studio Code, Sublime Text, and Atom provide a conducive environment for writing and managing code.
- Version Control Systems: Git, along with platforms like GitHub and GitLab, is essential for version control and collaboration.
- Design Tools: Applications like Adobe XD, Sketch, and Figma are used for creating and prototyping designs.
- Browser Developer Tools: Built-in tools in browsers like Chrome DevTools help debug and optimize web applications.
- Advanced Frameworks and Libraries: Tools such as Next.js for React, Nuxt.js for Vue.js, and SvelteKit for Svelte provide server-side rendering, static site generation, and other advanced capabilities.
- State Management Libraries: For complex applications, libraries like Redux for React or Vuex for Vue.js help manage application state more efficiently.
Backend Development: The Backbone of Web Applications
1. Server-Side Logic and Database Management
Backend development, or server-side development, handles the behind-the-scenes processes that power the frontend. This involves managing server, application, and database operations.
- Languages and Tools: Backend development typically involves languages like Python, Ruby, PHP, Java, and Node.js. These languages are used in conjunction with frameworks such as Django, Ruby on Rails, Laravel, and Express.js to build robust and scalable applications.
# Sample Python code block (using Flask)
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
- Database Management: Backend developers are responsible for managing databases, which store the data necessary for a website's operation. Common database management systems include MySQL, PostgreSQL, MongoDB, and SQLite.
2. Security and Authentication
One of the critical roles of backend development is ensuring the security of the web application. This includes implementing authentication and authorization protocols, data encryption, and other security measures to protect sensitive information.
- User Authentication: Backend developers create systems for user authentication, such as login functionalities, password management, and session handling.
// Sample Node.js code block (using Express.js)
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
- Data Protection: Ensuring data integrity and protecting against breaches are paramount. Backend developers implement encryption, secure APIs, and compliance with data protection regulations.
3. Resources Required for Backend Development
- Code Editors and IDEs: Tools like PyCharm, IntelliJ IDEA, and VS Code support backend development with various language-specific features.
- Database Management Tools: Tools like MySQL Workbench, pgAdmin, and Robo 3T (for MongoDB) help manage databases efficiently.
- Server Management: Services like AWS, Azure, and DigitalOcean provide scalable server solutions. Tools like Docker and Kubernetes help manage containers and orchestration.
- API Development: Postman is widely used for testing APIs, and Swagger helps with API documentation.
- Advanced Databases and Tools: Tools like Redis for in-memory data structure storage, Elasticsearch for search and analytics, and GraphQL for flexible APIs provide enhanced capabilities for complex applications.
- CI/CD Pipelines: Continuous Integration and Continuous Deployment tools like Jenkins, CircleCI, and Travis CI automate testing and deployment processes.
The Interdependence of Frontend and Backend
While frontend and backend development involve distinct skill sets and responsibilities, they are deeply interconnected. A successful web application relies on seamless communication between the frontend and backend.
- APIs and Data Exchange: The frontend communicates with the backend via APIs (Application Programming Interfaces). These APIs enable the exchange of data, allowing the frontend to present dynamic content retrieved from the server.
// Sample JSON response from an API
{
"status": "success",
"data": {
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com"
}
}
- Collaborative Development: Effective collaboration between frontend and backend developers is crucial. This includes coordinating on data structure, ensuring consistent data flow, and addressing issues related to compatibility and integration.
Conclusion
In the realm of web development, both frontend and backend development are essential. Frontend development focuses on creating a visually appealing and user-friendly interface, while backend development ensures the robust functionality and security of the application. The synergy between these two domains results in cohesive, performant, and engaging web applications that meet user needs and business goals. Understanding the significance of each, the resources required, and how they work together, is fundamental for anyone looking to excel in web development.
Further Reading and Resources
- MDN Web Docs: Comprehensive resources on
HTML, CSS, and JavaScript.
- W3Schools: Tutorials and references for web development technologies.
- freeCodeCamp: Interactive learning platform for frontend and backend development.
- Codecademy: Online courses on various programming languages and development tools.
- Stack Overflow: Community-driven Q&A platform for developers.
- GitHub: Platform for version control and collaborative development.
- Dev.to: Community of developers sharing articles and tutorials.
- Next.js Documentation: Guide for using Next.js with React.
- Docker Documentation: Comprehensive guide to containerization with Docker.
- Kubernetes Documentation: In-depth resource for orchestrating containers with Kubernetes.
- AWS Documentation: Resources for using Amazon Web Services.
Table Of Content
- Frontend Development: The Face of the Web
- 1. User Interface and User Experience
- 2. Performance and Responsiveness
- 3. Resources Required for Frontend Development
- Backend Development: The Backbone of Web Applications
- 1. Server-Side Logic and Database Management
- Sample Python code block (using Flask)
- 2. Security and Authentication
- 3. Resources Required for Backend Development
- The Interdependence of Frontend and Backend
- Conclusion
- Further Reading and Resources